Image Regions of Interest#
oneIPL functions operate on entire images or regions of interest (ROIs) within images. An ROI is a rectangular area that may be some part of the image or the whole image. ROIs are defined by the size and offset from the image origin as shown in figure below. The origin of an image is the top left corner, with x values increasing from left to right and y values increasing downwards.
Image, ROI, and Offsets
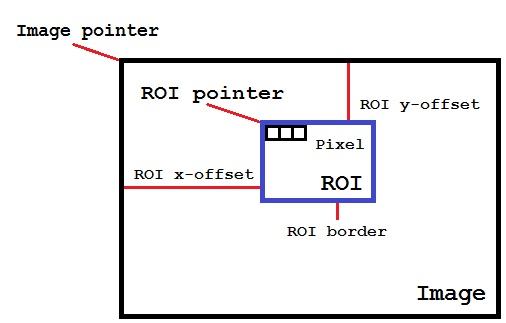
To fully describe a rectangular ROI, both its origin (coordinates of top left corner) and size must be referenced. The
ROI is specified by the roi_rect
parameter of ipl::image
constructor, meaning that all four values describing
the rectangular ROI are given explicitly.
-
struct roi_rect#
Class that represents a rectangle bounding a region of interest (ROI) of an image.
Public Functions
-
inline explicit roi_rect(const sycl::id<2> &offset, const sycl::range<2> &size)#
Constructor.
- Parameters:
offset – [in] the given 2D offset of ROI
size – [in] the given 2D size of ROI
-
inline roi_rect(const sycl::range<2> &size)#
Constructor of ROI rectangle that has a zero 2D offset.
- Parameters:
size – [in] the given 2D size of ROI.
-
inline std::size_t get_x_offset() const#
Returns the x offset.
- Returns:
the x offset
-
inline std::size_t get_y_offset() const#
Returns the y offset.
- Returns:
the y offset
-
inline std::size_t get_width() const#
Returns the width of the ROI (for the whole image returns the width of the image).
- Returns:
the width of the ROI
-
inline std::size_t get_height() const#
Returns the height of the ROI (for the whole image returns the height of the image).
- Returns:
the height of the ROI
-
inline explicit roi_rect(const sycl::id<2> &offset, const sycl::range<2> &size)#
Both source and destination images may have an ROI. If a function does not change the image size, the ROIs sizes are
assumed to be the same while the offsets may vary. Image processing is performed on the data of the source ROI, and the
results are written to the destination ROI. In the function call sequences, an ROI must be specified by the image
type constructed with the ROI parameter and can be obtained from the image object.
If an ROI is present:
the source and destination images map have different sizes
lines may have padding at the end for aligning the line sizes
Using ROI with Neighborhood
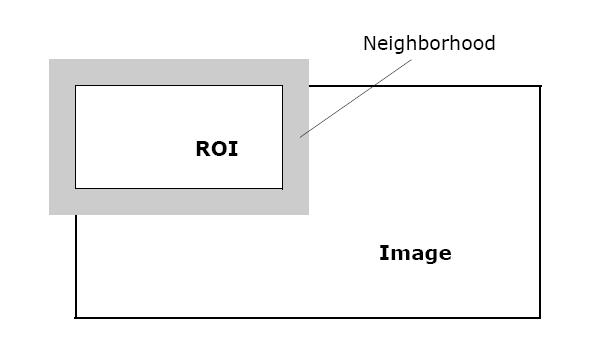
To ensure valid operation when image pixels are processed, the application should define the rules to handle border pixels, see Image Borders.
The code example below demonstrates how an image ROI can be used:
using namespace oneapi;
sycl::queue queue;
ipl::shared_usm_allocator_t allocator{ queue };
const sycl::range<2> image_size{ height, width };
const ipl::roi_rect dst_roi_rect{ image_size / 6, image_size / 3 };
// Source 4-channel image data and destination images
ipl::image<ipl::layouts::channel4, std::uint8_t> src_image{ queue, p_image_data, image_size, allocator };
// Gray image data
ipl::image<ipl::layouts::plane, std::uint8_t> gray_image{ image_size, allocator };
// Gray image ROI
ipl::image<ipl::layouts::plane, std::uint8_t> gray_image_roi = gray_image.get_roi(dst_roi_rect);
// Sobel image ROI
ipl::image<ipl::layouts::plane, std::uint8_t> sobel_image_roi{ gray_image_roi.get_range(), allocator };
// Run pipeline: convert to grayscale -> Sobel filter on ROI
(void)ipl::rgb_to_gray(queue, src_image, gray_image);
auto event = ipl::sobel(queue, gray_image_roi, sobel_image_roi);